Google analytics when implemented in a seemingly “standard” way is one of the common problems why websites do not get 100/100 scores in the Google PageSpeed test. Further below you’ll learn how to cleverly lazy load Google Analytics, so it does not impact these scores.
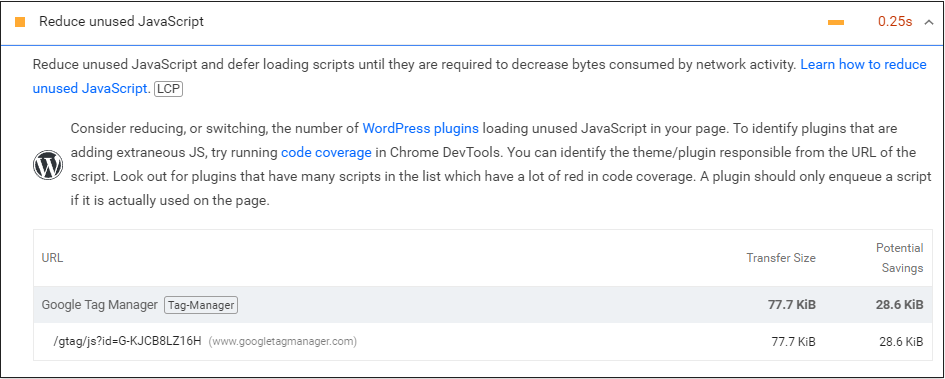
How to properly lazy load Google Analytics
The trick is actually to lazy load it in a bit different kind of way than usual (when all page contents has been loaded) – you must lazy load it on user interaction AFTER the DOM content has been loaded and the philosophy behind it is: You must not interrupt the process of rendering the page with optional stuff. Analytics is optional, page rendering is a priority.
A word of caution: With this method you should expect a bit lower visitor counts in Google Analytics, because not everyone who opens the page immediately interacts with it. At the same time you must ask yourself a question – are you really that interested in visitors which does not even scroll, tap or move a mouse cursor in your website? Well, I’m not, in favor of better Page Speed. 🙂
Here is the code that you should add just before the </body> closing tag. It will load Google Analytics once the site visitor has been interacted with page in one of the following ways: Click, Scroll, Mouse Move, Touch Start or if that does not happen – it will auto-load the Analytics 5 seconds after the page has been fully loaded.
<script>
var AnalyticsIsLoaded = false;
window.dataLayer = window.dataLayer || [];
function gtag(){dataLayer.push(arguments);}
gtag('js', new Date());
gtag('config', 'G-YOURTRACKINGID');
//Start dealing with analytics only when a DOM content has been fully loaded
document.addEventListener('DOMContentLoaded', function() {
//When the DOM content has been loaded, initialize analytics only on user interaction
['click', 'scroll', 'mousemove', 'touchstart'].forEach(function(e) {
window.addEventListener(e, InitAnalytics, {
once: true
});
});
function InitAnalytics() {
if (!AnalyticsIsLoaded) {
AnalyticsIsLoaded = true;
var e=document.createElement("script");
e.async=!0,e.src="https://www.googletagmanager.com/gtag/js?id=G-YOURTRACKINGID";
var a=document.getElementsByTagName("script")[0];a.parentNode.insertBefore(e,a);
}
}
setTimeout( function() {
InitAnalytics();
}, 6500); //If user has not interacted load analytics after 6.5 sec (to avoid false positives in speed metrics)
}, false);
</script>
Additional thing which you should adjust with this method is Google Analytics events. You must check if a gtag variable is defined, before triggering a Google Analytics event.
if (typeof gtag !== 'undefined') {
gtag('event', 'My Event', {'event_category' : 'Event Category', 'event_label' : 'Event Label'});
}